این سنسور دما رو به صورت 8 پیکسل خطی اندازه گیری میکنه و از طریق پروتوکل I2C به آردوینو ارسال میکنه
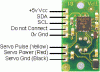
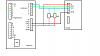
برای راه اندازی سنسور tpa81 از کد زیر استفاده کنید
کد:
#include <Wire.h>
#include <SoftwareSerial.h>
#define ADDRESS 0x68 // Address of TPA81
#define SOFTREG 0x00 // Byte for software version
#define AMBIANT 0x01 // Byte for ambiant temperature
int temperature[] = {0,0,0,0,0,0,0,0}; // Array to hold temperature data
void setup(){
Serial.begin(9600); // Starts software serial port for LCD03
Wire.begin();
delay(100); // Wait to make sure everything is powerd up
byte software = getData(SOFTREG); // Get software version
Serial.print("TPA81 Example V:");
Serial.print(software); // Print software version to the screen
}
void loop(){
for(int i = 0; i < 8; i++){ // Loops and stores temperature data in array
temperature[i] = getData(i+2);
}
for(int x = 0; x < 8; x++){ // Loop prints each member of temperature to LCD03 followed by a space
if(x==4) // If x is 4 perform a carriage return to format the results on the LCD03 for easier reading
Serial.print(temperature[x]);
Serial.print(" ");
delay(50); // Wait befor printing next value to give time for everything to be sent
}
int ambiantTemp = getData(AMBIANT); // Get reading of ambiant temperature and print to LCD03 screen
Serial.print("Ambiant: ");
Serial.print(ambiantTemp);
}
byte getData(byte reg){ // Function to receive one byte of data from TPA81
Wire.beginTransmission(ADDRESS); // Begin communication with TPA81
Wire.write(reg); // Send reg to TPA81
Wire.endTransmission();
Wire.requestFrom(ADDRESS, 1); // Request 1 byte
while(Wire.available() < 1); // Wait for byte to arrive
byte data = Wire.read(); // Get byte
return(data); // return byte
}